Integrating Routefusion with our new GraphQL API
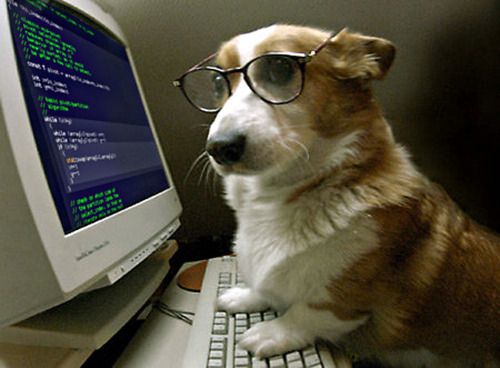
Unfolding payments in a simple GraphQL API.
Summary
This walkthrough will show you how to implement the routefusion API for products looking to deliver a 100% self-serve workflow to their customers to unlock cross-border payments. The implementation guide will take you through the execution of each workflow required will look like:
- Create an entity
- Create a Beneficiary
- Create a Transfer
- Get a rate (optional)
- Finalize the Transfer
Authentication
We will send you an API key in the form of a Bearer token which will need to be added as an Authorization
header in every network call to Routefusion.
curl --location --request POST '<https://sandbox.external.routefusion.com/graphql>' \\
--header 'Content-Type: application/json' \\
--header 'Authorization: Bearer yourBearerToken' \\
--data '{ someQuery }'
Create an entity
Now that the user has been created on routefusion we need to create the entity that is ultimately making the payment.
mutation createEntity($user_id: UUID!) {
createBusinessEntity(
user_id: $user_id
email: "developer@routefusion.com"
phone: "5125468912"
contact_first_name: "john"
contact_last_name: "smith"
business_name: "routefusion"
business_address1: "1305 E 6th"
business_address2: "unit 10"
business_city: "autin"
business_state_province_region: "tx"
business_postal_code: "78702"
business_country: "US"
business_type: "financial technology"
accept_terms_and_conditions: true
)
}
Create a beneficiary
Now that you have a user and an entity, you can go ahead and create a beneficiary (the recipient of the payment)
mutation createBeneficiary($user_id: UUID!, $entity_id: UUID!) {
createPersonalBeneficiary(
user_id: $user_id
entity_id: $entity_id
currency: "USD"
country: "US"
address1: "1234 5th Ave."
city: "austin"
first_name: "Jorge"
last_name: "Posada"
email: "email@test.com"
bank_country: "US"
bank_name: "Chase Bank"
swift_bic: "CHASUS33XXX"
account_number: "1234567"
routing_code: "0987654321"
)
}
Create a transfer
Once the user is approved and they have created beneficiaries, they can create their first transfer!
A little more on transfers first
Initiating a transfer is broken out into multiple steps in order to support the workflow which best fits the experience you want to deliver to your clients.
First, you will create a transfer. Once you have created the transfer, you can make changes to the transfer object, such as fetching a new rate, updating amounts, etc. Once the transfer is ready, you will finalize it, and you're done!
Transfers contain payment data (amounts and currencies), beneficiary data (entity and banking information), and rate data (exchange rate information, also known as a quote)
mutation createTransfer {
createTransfer(
user_id: "7a8546e6-f679-437e-8637-66c5875e5c7d"
entity_id: "96226ada-e7d9-46b0-9752-e401eb004997"
destination_amount: "50000.00"
source_currency: "USD"
beneficiary_id: "a9d0164d-22df-46c6-a836-1a3da5a470d8"
purpose_of_payment: "Goods and Services"
reference: "Invoice #201"
)
}
Get a quote
Once you have created a transfer, you can set a rate on the transfer which will lock in that rate for the transfer if you chose to finalize it. Rates will typically hold for 30 seconds. Once you finalize the transfer the rate will be locked.
mutation getTransferQuote {
getTransferQuote(
transfer_id: "a755b3e3-ac1b-4582-b0bb-b3b27a26c6b6"
) {
rate
inverted_rate
}
}
Finalize transfer
Now that the transfer has the desired data you are ready to initiate the payment! Finalizing the transfer will initiate the transfer to the beneficiary's bank account. This will lock in the rate you previously set on the transfer in the Get a quote section.
mutation finalizeTransfer ($transfer_id: UUID!) {
finalizeTransfer (transfer_id: $transfer_id)
}
You're done!
You nailed it! You have successfully sent your first payment with Routefusion 🙌
This will be a living document and may be updated. If you have any questions, please reach out and ask. If you have any suggestions we would love to hear them!