How to create recurring payments with Google Cloud Functions and the Routefusion API
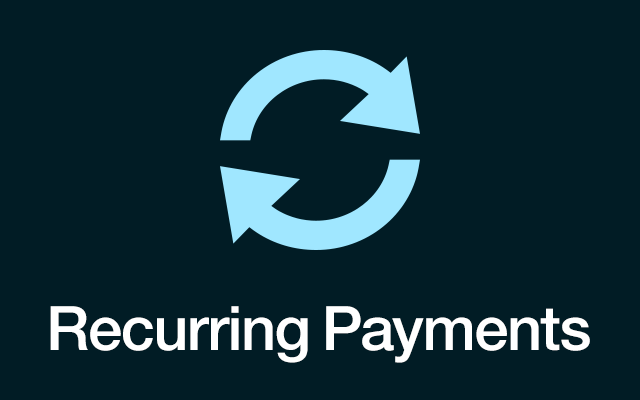
Ciao! At Routefusion we love programmable money. So today, to start the new year, we are going to do a brief tutorial on how you can create recurring payments using Google Cloud functions and the Routefusion API!
This tutorial will assume you already have a GCP account and project setup, with billing attached.
It will assume you also have the gcloud
command-line tools installed.
It will also assume that you have signed up on Routefusion and copied down your developer keys, created a beneficiary and have that beneficiary_id.
This tutorial will assume a few things:
- you already have a GCP account and project setup, with billing attached
- you also have the
gcloud
command-line tools installed. - you have signed up on Routefusion and copied down your developer keys, created a beneficiary and have that beneficiary_id
If you need to create GCP account, you can setup an account here https://cloud.google.com/, and if you do some searching around you will find out how you can get lots of free credits.
If you need to create a Routefusion account you can sign up here at routefusion.com.
If you need to download gcloud
command line tools you can do that here https://cloud.google.com/sdk/docs/install
Step 1: Create your repo and file structure, download the routefusion SDK.
Open a terminal, create a new directory called cron-send-payment
, and run npm init.
> mkdir cront-send-payment
> cd cron-send-payment
> npm init
Hit enter through the instructions for npm init, and then check out your file structure.
> ls
It should have only created you a package.json
Now create your index.js file
> touch index.js
Next, install the routefusion node SDK
> npm install --save routefusion-sdk
Step 2: Create your function
The next thing we are going to do is open our favorite code editor and create our Routefusion function. For ease you can just copy the code I wrote ;).
// index.js
// Initialize routefusion-sdk
const rf = require('routefusion-sdk').Instance({
RF_CLIENT_ID: process.env.RF_CLIENT_ID,
RF_SECRET: process.env.RF_SECRET,
RF_BASE_URL: process.env.RF_BASE_URL // will default to sandbox
});
// Create a cloud function that takes in stream data
exports.CreateTransferRoutefusion = (req, res) => {
// Decode the stream data into JSON
const PubSubData = JSON.parse(Buffer.from(req.data, 'base64')
// Create our transfer object
// developer.routefusion.co
const transferData = {
beneficiary_id: PubSubData.beneficiary_id,
source_amount: PubSubData.transfer_amount,
auto_complete: true,
source_currency: "USD"
};
// Make our recurring payment!
rf.createTransfer(transferData)
.then(resp => {
console.log(resp);
})
.catch(err => {
console.log(err)
})
};
A quick rundown of this function. We are initializing the Routefusion SDK
We then create a cloud function named CreateTransferRoutefusion
We JSON'ify (if thats a word) the data, and create our transfer object. Then we make our recurring payment! Super simple 😉
Step 3: Create your environment variables
You will notice that we have environment variables set. With cloud functions all you need to do is create a .env.yaml
file in project directory and add in your ENV vars.
> touch .env.yaml
Open your editor and paste in the below variables. Be sure to change the credentials to the ones found in your Routefusion developer tab on routefusion.com.
# .env.yaml
RF_CLIENT_ID: 0C270CB98CFD4DE4BBD45BA179B67307F263DA92C66572A2A8D043E55FC826B5
RF_SECRET: 723Ae7657b97722DA2D4a964EB1D137d21B4A81e88AF368F1e990B8b2F5727C0
RF_BASE_URL: <https://sandbox.api.routefusion.co>
Step 4: Quick cleanup of directory
For good measure lets create a .gcloudignore.
> touch .gcloudignore
Open your editor and paste this in .gcloudignore
# .gcloudignore
# This file specifies files that are *not* uploaded to Google Cloud Platform
# using gcloud. It follows the same syntax as .gitignore, with the addition of
# "#!include" directives (which insert the entries of the given .gitignore-style
# file at that point).
#
# For more information, run:
# $ gcloud topic gcloudignore
#
.gcloudignore
# If you would like to upload your .git directory, .gitignore file or files
# from your .gitignore file, remove the corresponding line
# below:
.git
.gitignore
node_modules
Step 5: Create your gcloud pubsub topic
If you are not familiar with PubSub, take some time and read about it here. https://blog.stackpath.com/pub-sub/
Ok, lets create our topic. Open your terminal and type the following
> gcloud pubsub topics create create-transfer
Wow, that was easy! Now make sure you topic was created
> gcloud pubsub topics list
It should give you output that looks like this
> gcloud pubsub topics list
---
name: projects/YOUR_PROJECT_NAME/topics/create-transfer
Sweet! Now lets create our cloud function.
Step 6: Create your cloud function
Similar to creating a topic we use the gcloud
command line tools. Super convient. You can find a list of all the gcloud function
commands here for reference. https://cloud.google.com/sdk/gcloud/reference/functions/deploy
From your terminal with a working directory inside of your directory we setup earlier run the below command.
> gcloud functions deploy CreateTransferRoutefusion \\
--trigger-topic create-transfer --runtime nodejs10 \\
--env-vars-file .env.yaml
After the command runs maker sure it was created by running the below command
> gcloud functions list
NAME STATUS TRIGGER REGION
CreateTransferRoutefusion ACTIVE Event Trigger us-central1
Great, now our function has been created! Now for the last part.
Step 7: Create your a cron job to send recurring payments
For this tutorial we are going to automate the paying of our rent every month. Earlier at the start of the tutorial we mentioned we are assuming you have created a beneficiary. Make sure you have that beneficiary id, and lets get our function live!
From your terminal run the below command.
> gcloud scheduler jobs create pubsub PayRentMonthly \\
--schedule "0 0 1 * *" --topic create-transfer \\
--message-body "{"beneficiary_id": YOUR_BENEFICIARY_ID, "transfer_amount": YOUR_RENT_AMOUNT }"
That command creates a cron job that will publish a message with the transfer details on the first of every month.
Just to double check that it was created lets run the scheduler command
> gcloud scheduler jobs list
ID LOCATION SCHEDULE (TZ) TARGET_TYPE STATE
PayRentMonthly us-central1 0 0 1 * * (America/Chicago) Pub/Sub ENABLED
Step 8: Sit back and relax, you will never forget to pay your rent again!
I hope you enjoyed this tutorial. If you want this payment to go live, all you need to do is submit compliance documents on routefusion.com. We will approve your account for production access ASAP. Once you are approved you will link a bank account and everything will just work! How fun is that.
If you have any questions or want to discuss any cool ideas you have for programming money, please email the team at engineering@routefusion.com
. We love to chat about all sorts of ideas and problems.
Happy Coding!